With over 700,000 apps in App Store, your app must have a great design to stand out from others. If your app is still using the default design, you better give it a tweak and make it look better. I’m sure it’ll improve your app sales and attract more downloads.
When you check out any popular app, you’ll notice a custom design tab bar. Some readers asked how we can change the appearance of the tab bar. In this tutorial, we’ll show you how to do that. With the introduction of Appearance API in iOS 5, it’s much easier to custom the UI controls that gives your app a unique look and feel.
An appearance proxy is an object you use to modify the default appearance of visual objects such as views and bar items. Classes that adopt the UIAppearance protocol support the use of an appearance proxy. To modify the default appearance of such a class, retrieve its proxy object using the appearance class method and call the returned object’s methods to set new default values. A proxy object implements those methods and properties from its proxied class that are tagged with the UI_APPEARANCE_SELECTOR macro. For example, you can use a proxy object to change the default tint color (through the progressTintColor or trackTintColor properties) of the UIProgressView class.
As usual, we’ll build a sample app to walk you through the customization. The app doesn’t have any features but our primary focus here is to show you how to apply the Appearance API and change the appearance of tab bar including the background image and title color. We’ll also modify the images of UITabBarItem as well. Let’s first take a look at the final deliverable.
Understanding UITabBar and UITabBarItem
Before we dig into the API, let’s first take a look at how the UITabBar and UITabBarItem are designed:
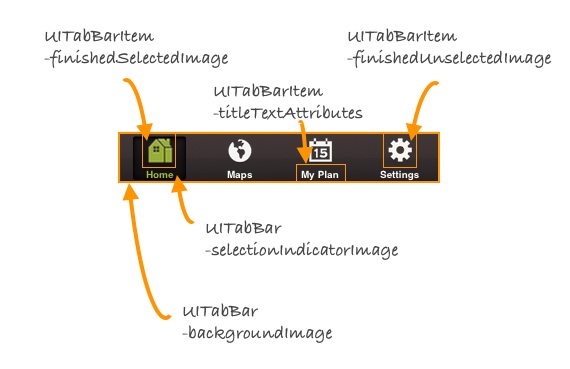
UITabBar and UITabBarItem Appearance
The above illustration should give you a better idea how you can customize the UI controls. Now let’s start to build the sample app.
Create a Sample Tab Bar App
First, create a new Xcode project using the “Tabbed Application” Template. Let’s name the project as “CustomTabApp” and set the project with the following parameters:
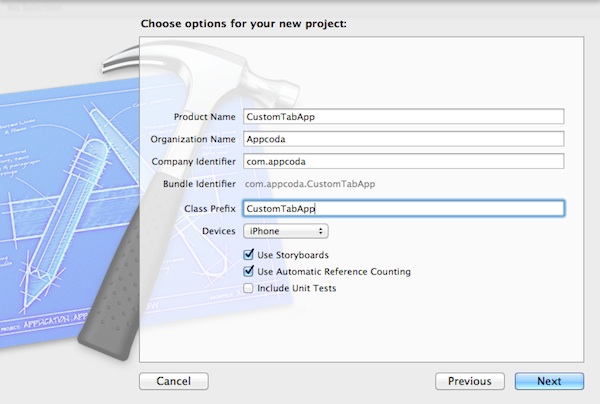
CustomTabApp – New Project
After Xcode created the project, download this image set and add all the images file to your Xcode project.
Add More Tabs to Tab Bar Controller
Go to Storyboard and design the interface. As the project is first generated, it comes with two tab bar items only. Let’s add two more. (Note: If you’re new to tab bar controller, check out our earlier tutorial about how to create a tab bar controller using Storyboard.)
Simply add two view controllers and associate them with the Tab Bar Controller. Press and hold the control key, click the Tab Bar Controller and drag it towards the new view controllers. Select “view controllers” for Relationship Segue.
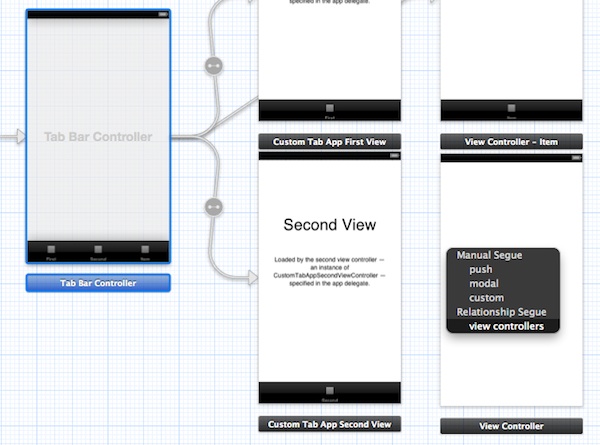
Associates Two More View Controllers with the Tab Bar Controller
Once done, you should have a Tab Bar Controller with 4 tabs. Run the app and this is how it looks like:

CustomTabApp with 4 Tabs
Change Title and Icon of the Tab Bar Items
Next, select the “CustomTabAppAppDelegate.m” and edit the “didFinishLaunchingWithOptions” method as below:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
// Assign tab bar item with titles
UITabBarController *tabBarController = (UITabBarController *)self.window.rootViewController;
UITabBar *tabBar = tabBarController.tabBar;
UITabBarItem *tabBarItem1 = [tabBar.items objectAtIndex:0];
UITabBarItem *tabBarItem2 = [tabBar.items objectAtIndex:1];
UITabBarItem *tabBarItem3 = [tabBar.items objectAtIndex:2];
UITabBarItem *tabBarItem4 = [tabBar.items objectAtIndex:3];
tabBarItem1.title = @"Home";
tabBarItem2.title = @"Maps";
tabBarItem3.title = @"My Plan";
tabBarItem4.title = @"Settings";
[tabBarItem1 setFinishedSelectedImage:[UIImage imageNamed:@"home_selected.png"] withFinishedUnselectedImage:[UIImage imageNamed:@"home.png"]];
[tabBarItem2 setFinishedSelectedImage:[UIImage imageNamed:@"maps_selected.png"] withFinishedUnselectedImage:[UIImage imageNamed:@"maps.png"]];
[tabBarItem3 setFinishedSelectedImage:[UIImage imageNamed:@"myplan_selected.png"] withFinishedUnselectedImage:[UIImage imageNamed:@"myplan.png"]];
[tabBarItem4 setFinishedSelectedImage:[UIImage imageNamed:@"settings_selected.png"] withFinishedUnselectedImage:[UIImage imageNamed:@"settings.png"]];
return YES;
}
The above code is very straightforward but let me explain the code line by line.
Line 4-9 – As we use the Tabbed Application Xcode template, the UITabBarController is assigned as the root view controller. From the tabBarController, we can retrieve each individual tab item.
Line 11-14 – Assign the title of the tab bar items
Line 16-19 – Set the images of the tab bar items. There are two types of images: FinishedSelectedImage and FinishedUnselectedImage. When user selects a tab item, the selected tab item will be highlighted with the “FinishedSelectedImage”, while the rest of the tab items will display the “FinishedUnselectedImage”.
When you run the app again, this is how it looks like:

CustomTabApp – Tabs with Icons and Titles
Styling the Tab Bar
Next, we’ll change the background image of the entire tab bar and the selection indicator. As said before, the Appearance API makes it very easy to customize the background. Add the following code to the “didFinishLaunchingWithOptions” method:
UIImage* tabBarBackground = [UIImage imageNamed:@"tabbar.png"];
[[UITabBar appearance] setBackgroundImage:tabBarBackground];
[[UITabBar appearance] setSelectionIndicatorImage:[UIImage imageNamed:@"tabbar_selected.png"]];
The code tells your app to use “tabbar.png” as the background image of tab bar (The dimension is 320 x 49 pixels) globally. We also update the selection indicator image that better fits with the new background.
Run the app again and you should now get a tab bar with better look and feel:

CustomTabApp with New Tab Bar Background
Changing the Text Color of Tab Bar Item
By default, the title color for the unselected tabs is in gray while the selected one is in white. To change the color we alter a dictionary of attributes with the UITextAttributeTextColor key:
[[UITabBarItem appearance] setTitleTextAttributes:[NSDictionary dictionaryWithObjectsAndKeys:
[UIColor whiteColor], UITextAttributeTextColor,
nil] forState:UIControlStateNormal];
UIColor *titleHighlightedColor = [UIColor colorWithRed:153/255.0 green:192/255.0 blue:48/255.0 alpha:1.0];
[[UITabBarItem appearance] setTitleTextAttributes:[NSDictionary dictionaryWithObjectsAndKeys:
titleHighlightedColor, UITextAttributeTextColor,
nil] forState:UIControlStateHighlighted];
Add the above code to the “didFinishLaunchingWithOptions” method. For unselected tabs (i.e. with state of “UIControlStateNormal”), we change the text color to white. For selected tabs, we want to have a special green color that can’t be found in the UIColor class. Therefore, we initialize a new color object with specific color code (i.e. red: 153, green: 192, blue: 48).
That’s it! The final step is to run the app and check out the result. You should now have a fully customized tab bar.

CustomTabApp – Fully Customized Tab Bar
Complete Code
For your easy reference, here is the complete code for the customization:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
// Assign tab bar item with titles
UITabBarController *tabBarController = (UITabBarController *)self.window.rootViewController;
UITabBar *tabBar = tabBarController.tabBar;
UITabBarItem *tabBarItem1 = [tabBar.items objectAtIndex:0];
UITabBarItem *tabBarItem2 = [tabBar.items objectAtIndex:1];
UITabBarItem *tabBarItem3 = [tabBar.items objectAtIndex:2];
UITabBarItem *tabBarItem4 = [tabBar.items objectAtIndex:3];
tabBarItem1.title = @"Home";
tabBarItem2.title = @"Maps";
tabBarItem3.title = @"My Plan";
tabBarItem4.title = @"Settings";
[tabBarItem1 setFinishedSelectedImage:[UIImage imageNamed:@"home_selected.png"] withFinishedUnselectedImage:[UIImage imageNamed:@"home.png"]];
[tabBarItem2 setFinishedSelectedImage:[UIImage imageNamed:@"maps_selected.png"] withFinishedUnselectedImage:[UIImage imageNamed:@"maps.png"]];
[tabBarItem3 setFinishedSelectedImage:[UIImage imageNamed:@"myplan_selected.png"] withFinishedUnselectedImage:[UIImage imageNamed:@"myplan.png"]];
[tabBarItem4 setFinishedSelectedImage:[UIImage imageNamed:@"settings_selected.png"] withFinishedUnselectedImage:[UIImage imageNamed:@"settings.png"]];
// Change the tab bar background
UIImage* tabBarBackground = [UIImage imageNamed:@"tabbar.png"];
[[UITabBar appearance] setBackgroundImage:tabBarBackground];
[[UITabBar appearance] setSelectionIndicatorImage:[UIImage imageNamed:@"tabbar_selected.png"]];
// Change the title color of tab bar items
[[UITabBarItem appearance] setTitleTextAttributes:[NSDictionary dictionaryWithObjectsAndKeys:
[UIColor whiteColor], UITextAttributeTextColor,
nil] forState:UIControlStateNormal];
UIColor *titleHighlightedColor = [UIColor colorWithRed:153/255.0 green:192/255.0 blue:48/255.0 alpha:1.0];
[[UITabBarItem appearance] setTitleTextAttributes:[NSDictionary dictionaryWithObjectsAndKeys:
titleHighlightedColor, UITextAttributeTextColor,
nil] forState:UIControlStateHighlighted];
return YES;
}
What’s Next?
In this tutorial, we show you the basics about how to change the appearance of tab bar using Appearance API. If you’ve already developed an app or you have completed the Restaurant App tutorial, go ahead to tweak the app and make your own tab bar.
As always, feel free to leave comment here or in our forum if you have any questions.